Where to put the code
Any time you add a custom PHP function to your site, insert it in the WPCode plugin for code snippets (recommended), a child theme's functions.php, or your custom plugin.
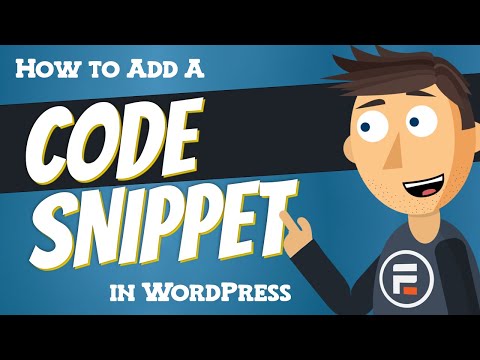
If you insert PHP code using the WPCode plugin, ensure that the PHP Snippet is selected as the code type.
If you're unfamiliar with using WordPress filters, you can find more details in the WordPress docs.
Duplicating a function
If you are attempting to use the same function or hook multiple times, you can't just duplicate the code and change field ids. Using the same function name twice will result in fatal errors on your site. To avoid this issue, you must change the function name any time you use the same code more than once on your site.
The function name is typically in two places within the code and needs to be changed in both places it occurs. In this example, the function name "check_entry_count" would need to be changed to something unique like "check_entry_count2" or "check_formb_entry_count". As long as the function names are unique, you can use the same custom code multiple times.
add_action('frm_display_form_action', 'check_entry_count', 8, 3); function check_entry_count($params, $fields, $form){ global $user_ID; remove_filter('frm_continue_to_new', '__return_false', 50); if($form->id == 11 and !is_admin()){ //replace 5 with the ID of your form $count = FrmEntry::getRecordCount("form_id=". $form->id ." AND user_id=".$user_ID); if($count >= 1){ //change 2 to your entry limit echo 'This form is closed'; add_filter('frm_continue_to_new', '__return_false', 50); } } }
Form Appearance
frm_scroll_offset: After a form is submitted, it scrolls to the success message or first error message. With some themes, this may be underneath a floating header or otherwise misplaced. Use this hook to add extra offset to the scrolling.
formidable_shortcode_atts: Use custom parameters in the [formidable] shortcode.
frm_jquery_ui_base_url: Some jQuery scripts and styles are loaded from the external Google API (ajax.googleapis.com/ajax/libs/jqueryui/####). This hook allows this URL to be changed. Files loaded from this URL includes:
- The jQuery style selected in the form styling settings
- The jQuery script or datepicker localization
frm_setup_new_form_vars: Change the default values used to create a new form.
frm_success_filter: This hook can be used to manipulate a form's success message.
frm_form_error_class: This hook allows you to add CSS classes to the validation message class for all forms.
frm_message_placement: Move the form success message below the form. If this position will always be the same for a single form, you can add a form class to avoid custom code.
frm_run_honeypot: Use this hook to turn off Honeypot.
frm_global_login_msg: This hook can be used to change the login message in the global settings.
frm_global_failed_msg: This hook can be used to customize the failed/duplicate entry message in the Formidable → Global settings → Message Defaults. It is the "We're sorry. It looks like you've already submitted that" message.
frm_global_invalid_msg: This hook can be used to change the incorrect field message in the global settings.
frm_global_setting: This hook can be used to filter global setting values.
frm_form_strings: This hook can be used to get a list of all form string settings.
frm_fields_in_form_builder: This hook allows you to modify the list of fields in the form builder.
frm_fields_in_settings: This hook allows you to modify the list of fields in the form settings.
frm_images_dropdown_output: This hook allows you to modify the output of the FrmAppHelper::images_dropdown() method.
frm_images_dropdown_input_attrs: This filter allows to modify the HTML attributes of radio inputs in FrmAppHelper::images_dropdown() method.
frm_images_dropdown_option_image: This hook allows to modify the HTML image of each option in FrmAppHelper::images_dropdown() method.
frm_images_dropdown_option_classes: This hook allows to modify the CSS classes of each option in FrmAppHelper::images_dropdown() method.
frm_images_dropdown_option_html_attrs: This hook allows to modify the custom HTML attributes of each option in FrmAppHelper::images_dropdown() method.
frm_submit_button_html: This hook allows you to change the HTML of the submit button before it applies the custom submit button classes.
frm_disallow_unfiltered_html: This hook is only used if the DISALLOW_UNFILTERED_HTML constant is NOT set. If the DISALLOW_UNFILTERED_HTML constant is set, it will always be disallowed.
- It adds another way to disallow unfiltered HTML in cases where setting DISALLOW_UNFILTERED_HTML might not be ideal. e.g. users might want to allow unfiltered HTML in WordPress or other plugins but not in Formidable.
- When unfiltered HTML is disallowed, it means that we will run kses on it. Script tags will not work, as well as other unexpected HTML that could be possibly unsafe but plenty of HTML will continue to work even when HTML is being filtered.
- This filter only applies to HTML that is rendered by Formidable, unlike DISALLOW_UNFILTERED_HTML which applies to the entire site.
frm_pro_default_form_settings: This filter allows you to change the Formidable Forms Pro default settings.
frm_chat_progress_text: This hook allows you to customize the progress bar/text in conversational forms. The progress text can be changed from the default Question [current] of [total] to something custom. It also supports [current show=percent].
frm_chat_start_page_content: This hook allows you to customize the conversational form start page.
frm_form_enctype: Use this hook to set a custom enctype for a form.
frm_use_custom_header_ip: By default, the Use custom headers when retrieving IPs with form submissions option in the Formidable → Global Settings page is hidden when the GDPR IP Storage option is enabled. Use this filter to enable this option and use the GDPR setting while behind a reverse proxy.
frm_conf_input_backend: Use this to filter the HTML of the email or password confirmation field input in the form builder. This is useful if you want to modify the HTML of the confirmation field input in the front end and apply that change to the form builder in the back end.
frm_back_button_class: The frm_back_button_class filter allows you to add classes to a back or previous button.
frm_summary_email_content_args: Use this hook to filter the data passed to the summary email content.
frm_html_summary_emails: This filter handles the change between HTML and plain text summary emails. It applies to summary emails only.
frm_allowed_form_input_html: By default, an unprivileged user can only submit a few tag types with no attributes: p, br, strong, b, i, ul, ol, and li. You can use this filter if you want to add more. When Formidable Forms Pro is active, additional tags are allowed by default for better compatibility with rich text fields, including h1-h6, blockquote, pre, code, hr, del, and ins.
frm_display_form_action: This hook allows you to do something before the form is displayed, such as checking the number of entries and displaying a message if the number of entries has reached its limit.
frm_main_feedback: This hook can be used to replace the form success/update message with a custom success/update message.
frm_form_classes: This hook allows you to add classes to the <form> tag for all forms or only those that are specified. This code can be used to add a background color to your forms.
frm_submit_button_action: This hook allows you to add HTML to the submit button.
frm_submit_button: This hook allows you to change the label on all or some Submit buttons.
frm_form_fields_class: This hook allows you to easily add a class to the frm_form_fields div which surrounds your forms.
frm_ajax_load_styles: When a form is loaded with ajax, the scripts and styles are prevented from loading. If you have a style you would like loaded when a form is rendered with ajax, you can allow it with this hook.
frm_show_form_after_edit: The form settings include an option to show the form with the success message. This setting applies when editing an entry as well. If you would like the form to show differently after edit than it does after submit, use this hook.
frm_entry_form: This hook can be used to add anything, such as a field, to a form. By default, any additions will not be saved with the form entry.
frm_filter_final_form: This hook can be used to make changes to the rendered form content before it is returned by the form shortcode.
frm_entries_footer_scripts: This hook allows you to add javascript to the footer of your form. You can also add javascript in your form Settings → Customize HTML in the before or after fields.
get_frm_stylesheet: Use this hook to set a custom stylesheet for your forms.
Field Appearance
frm_repeat_start_rows: Use this hook to determine how many rows should appear in a repeating field when the form is initially loaded.
frm_radio_class: This hook allows you to add classes to a radio field container on the .frm_radio div.
frm_date_field_options: Alter the datepicker options before they are converted to javascript.
frm_add_form_style_class: Each form style will have its own unique class name. When this form class name is added to the form, it will allow control for which styling settings the form uses. This hook will allow you to select a different style than the one already selected in the form settings.
frm_allow_date_mismatch: This hook can be used to allow different date formats in your form.
frm_currency: The currency for pricing fields is set in the Global Settings for all forms. If some forms should use a different currency than others, use this hook.
frm_fields_in_tags_box: This hook allows you to modify the list of fields in the Customization panel tags box.
frm_radio_display_format_options: This hook allows modifying the options of display format setting for the radio or checkbox field.
frm_choice_field_option_label: This filter allows changing the HTML of the option label in the radio or checkbox field.
frm_fields_in_form: This filter allows modifying the list of fields in the form.
frm_include_dropzone_in_minified_js: Use this hook if you want the dropzone to be included separately from frm.min.js. It can be used to resolve a conflict that prevents dropzone uploads when a form is loaded on a BuddyBoss documents page. It won't happen immediately. You would need to run add_action( 'plugins_loaded', 'FrmAppHelper::save_combined_js' ); once to regenerate the JS (but you do not want to run that on every page load, just the first filter).
frm_include_maskedinput_in_minified_js: Use this filter to exclude jQuery Masked Input from frm.min.js. The maskedinput JavaScript is used for the phone fields and other fields with custom pattern rules. It can be used to resolve a known conflict with WooCommerce Stripe Gateway. It is now automatically detected, and it will load the maskedinput JavaScript separately to avoid conflict.
frm_focus_first_error: Use this filter to toggle off the behavior of the auto-focus that gets triggered on the first field with an error.
frm_include_alert_role_on_field_errors: Use this filter to toggle the alert role that gets added to field errors.
frm_section_is_open: Use this filter to conditionally load collapsible sections as open, open all collapsible sections, or a specific target section.
frm_pro_show_password_icons: Use this filter to show and hide the password icons.
frm_chosen_js: Use this filter to customize the options for Chosen, our autocomplete dropdown library.
frm_phone_pattern: Use this filter to define the phone field format for all fields.
frm_should_dynamic_field_use_option_label: If you're using a shortcode that retrieves data for a dynamic field with separated values, you can use this filter to pull the value instead of the label.
frm_use_chosen_js: By default, autocomplete dropdowns will use Slim Select, enabling autocomplete on mobile devices. If you prefer to revert to Chosen JS, you can switch back using this filter. It's important to note that after adding this filter, you should also regenerate the generated CSS and JavaScript files, or it may not load properly.
frm_custom_html: This hook allows you to change the default HTML for all newly created fields. It will not change HTML for existing fields.
frm_replace_shortcodes: Use this hook to change the HTML for any fields in your form.
frm_field_input_html: This hook can be used to add HTML within input tags in a field. It is especially helpful for adding HTML5 placeholder text, required attributes, and formatting field input.
frm_recaptcha_lang: This hook can be used to change the language of individual Google ReCaptcha fields.
frm_field_div_classes: This hook will allow you to add CSS classes to the div container surrounding a field. This div container's CSS id would be frm_field_x_container, where x is the field ID.
frm_field_classes: This hook allows you to add classes to an input field. Classes can be added without custom code by going into edit your customizable HTML, and changing [input] to [input class="my_class"].
frm_file_icon: This hook is used for altering the HTML for a file displayed with a file upload field when editing your form.
frm_load_dropzone: If you don't love the Dropzone uploader or are using a custom uploader, you can disable it. Using this hook does not block dropzone uploads from happening. For added security, use this code snippet to disallow uploads that could happen before the entry is created. It is done by blocking the Dropzone action and not loading the Dropzone.
frm_selectable_dates: This hook allows you to manipulate specific dates within a date field. NOTE: The date field must have the unique option selected.
This hook allows you to customize the pop-up datepicker calendar in a date field that does not use any options from the Datepicker Options plugin.
frm_datepicker_formats: This hook can be used to add a custom date format in your Global Settings for Date Formats. The first date format is the PHP date format, which is 'j M, Y' in the example below. Get more information on PHP date formats. The second date format will be the Datepicker format which is d M, yy. This format will control how the date is displayed in the Date field. Get more information on Datepicker compatible formats. The Datepicker date format must correspond to the appropriate PHP date format.
frm_get_paged_fields: If you have a multi-page form, you can use this filter to display the same field multiple times.
frm_rte_options: This hook will allow options to be limited or added to the TinyMCE editor of a Rich Text field.
frm_combo_dropdown_label: This hook can be used to change the labels in Address fields and Credit Card fields.
Field Options and Values
frm_user_id_display: This hook allows you to show something other than the display name by default in user ID fields
frm_field: This hook can be used to filter a form field. Note: The frm_field filter is only applied when FrmField::getOne is called with the $filter = true parameter set, which is not on by default. If you want to modify a field with a filter that gets applied by default, use frm_setup_new_fields_vars instead.
frm_field_value_object: This hook can be used for making adjustments to the field before it's label and value is displayed.
frm_currencies: This hook can be used to adjust the attributes of the available currencies in a form.
frm_images_dropdown_args: This hook allows to modify the arguments of FrmAppHelper::images_dropdown() method.
frm_radio_display_format_args: This hook allows modifying the arguments before passing them to the FrmAppHelper::images_dropdown() method.
frm_entry_values_include_fields: This hook allows modifying the included field IDs used in the entry values.
frm_entry_values_exclude_fields: This hook allows modifying the excluded field IDs used in the entry values.
frm_entry_values_fields: This hook allows modifying the list of all fields in the form that is used in the entry values.
frm_pro_fields_in_dynamic_selection: This filter allows modifying the list of fields in the dynamic selection of the Dynamic field.
frm_pro_fields_in_lookup_selection: This filter allows modifying the list of fields in the lookup selection of Lookup field.
frm_switch_field_types: This filter allows the switching of field types.
frm_pro_fields_in_summary_values: This filter allows you to change the fields that are included in a summary field.
frm_unique_field_key_separator: This hook can be used to generate unique field keys by allowing custom field key separators.
frm_unique_form_key_separator: This hook can be used to generate unique form keys by allowing custom form key separators.
frm_address_sub_fields: Use this filter to customize an address field, such as removing the second line of the street address. It can also be used to add new sub fields.
frm_dynamic_field_include_drafts: As of version 6.9, dynamic fields will only include data from submitted entries. Draft entries, in-progress entries, and abandoned entries will be ignored by default. Use this filter to modify the default behavior with support for the new options.
frm_new_form_values: Use this filter to customize the values of a new form that gets created with the new form pop up.
frm_autocomplete_options: Use this filter to customize the options shown in the new Autocomplete setting for Text type fields.
frm_prepare_data_before_db: This filter allows including a leading character in the entry value.
frm_restrict_options_for_logged_out_users: Dynamic fields can restrict options to those created by the logged-in user. However, when no user is logged in, all options (including those from other users) are displayed by default. Use this filter if you want all options to be available regardless of the user's login status.
frm_error_substrings_to_replace_with_field_name: By default, errors will replace This field and This value settings values dynamically with the field name. This filter allows you to:
- Only dynamically replace [field_name] shortcodes.
- Add additional substrings for replacement.
- Add additional translated strings, if you wanted to convert more substrings universally in a single place.
frm_should_search_admin_entries_created_at_in_utc: When searching for entries by “Entry creation date” or the updated timestamp on the entries list page, entries will now be done in the server timezone. Use this filter to revert to UTC searches.
frm_field_validation_include_data_attributes: Use this filter to include or exclude the data-invmsg/data-reqmsg attributes that are used for JS validation. By default, these will now be removed for hidden fields in v6.9+.
frm_setup_new_fields_vars: This hook can be used to change field values, options, and other field settings when creating an entry. Note: If you are using this hook to change the options in a dropdown field, these options will only be available when creating a new entry. The options added with this hook will not be available when updating a past entry. A dropdown field using this hook to populate its values will appear blank when editing the entry.
frm_setup_edit_fields_vars: This hook can be used to change field values, options, and other field settings when editing an entry.
frm_get_default_value: Set the default value of any field when your form is displayed. The default value will ONLY apply to new entries and will not be applied when editing an entry.
frm_field_type: Use this hook to hide fields depending on certain conditions.
frm_data_sort: This hook allows you to change the order of the options in a Dynamic field or remove unwanted options. This hook cannot be used for dependent Dynamic fields. Instead, use the frm_setup_new_fields_vars hook.
frm_bulk_field_choices: This hook can be used to add your own collection of Bulk Edit options for fields in your forms. These can be found when clicking "Bulk Edit Options" in a dropdown, radio, or checkbox field.
frm_get_categories: This hook can be used to alter the options returned in a Dynamic field that is mapped to a category/taxonomy.
This hook allows shortcodes in field values to be processed rather than shown on the page.
Added security protections prevents shortcodes inside a field from being processed in user-submitted data. If you would like to opt-out of this protection, you can use this filter.
frm_allowed_times: This hook allows you to gray out specific times in a time field. It will only apply when there is a date field in the form and the 'Unique' option is enabled in the Time field.
frm_set_comparison_type_for_lookup: This hook can be used to change the comparison operator for Lookup fields. By default, Lookup fields only retrieve values that are exactly equal to the selected/entered value.
frm_order_lookup_options: This hook allows you to modify the order of options in a Lookup field.
frm_filtered_lookup_options: Modify the options included in a lookup field before they are displayed in the form.
frm_show_it: Use this hook to customize the value displayed in a Dynamic List field (previously called a Dynamic - Show It field, hence the hook name).
frm_lookup_is_current_user_filter_needed:
Entry Management
frm_validate_field_entry: This hook can be used to create your own custom field validations and errors. The Pro validation is also run using this hook. It runs with a priority of 10. The Pro validation will remove any error messages for fields that are conditionally hidden, so you may want to run your function with a priority below 10. This hook is run every time the submit button is clicked.
frm_validate_entry: This hook allows you to add custom errors that are not specific to one field.
frm_after_create_entry: This hook allows you to do something with the data entered in a form after it is submitted. This is the best place for a custom action after form submit.
frm_after_update_entry: This hook allows you to do something with the data entered in a form after it is updated.
frm_entries_before_create: This hook allows you to add trigger actions and add errors after all other errors have been processed.
frm_check_blacklist: Disable the comment blacklist check on some or all forms.
frm_include_meta_keys: This hook makes it so when an entry object is retrieved, like this: $entry = FrmEntry::getOne( $id, true) then the $entry->metas includes the field values by ID and also by key. Normally when retrieving an entry object, the $entry -> metas would only include the field values by ID.
frm_entries_list_query: By default, all entries show on the Formidable -> Entries pages. This hook can be used to change which entries are included on the back-end listing pages.
frm_input_masks: This hook allows you to add an input mask.
frm_field_value_saved: This hook can be used to set a different input value before the form is displayed.
frm_show_delete_all: Show or hide the 'Delete ALL Entries' button with custom conditions.
frm_admin_search_options: This hook can be used to add options to admin entry search.
frm_filter_admin_entries: This hook can be used to customize the admin entries search.
frm_process_honeypot: This hook can be used to manually flag the submission of a form as honeypot spam.
frm_filename_spam_keywords: This hook can be used to validate that uploaded file is not obviously spam.
frm_process_antispam: This hook can be used to define new content inside a Business Directory suntab settings section in the WordPress admin.
frm_form_token_check_before_today: This hook can be used to extend the valid token times before current date. Learn more about Antispam Javascript token.
- It is a filter for extending the timeframe for our Check entries for spam using JavaScript setting. If this isn't enabled, this filter won't do anything.
- The error message that is associated with this filter is Form token is invalid. Please refresh the page.
frm_form_token_check_after_today: This hook can be used to extend the valid token times after current date. Learn more about Antispam Javascript token.
- It is a filter for extending the timeframe for our Check entries for spam using JavaScript setting. If this isn't enabled, this filter won't do anything.
- The error message that is associated with this filter is Form token is invalid. Please refresh the page.
frm_duplicate_check_val: This hook can be used to run filter on duplicate check values.
frm_max_filename_length: Long file names will be automatically truncated on upload for names longer than 100 characters. Use this hook to customize this limit.
frm_delete_temp_files_period: Use this hook to adjust the default -3 hours period before temporary files are deleted.
frm_fields_in_entries_list_table: This hook allows you to modify the list of fields in the entries list table.
frm_fields_to_validate: This hook is called before validation happens. If you exclude a field here, it should skip validation.
frm_after_duplicate_field: This action hook fires after duplicating a field.
frm_stop_file_switching: Use this hook to determine whether file protection will prevent file switching sitewide or only for specific forms where this option is enabled.
frm_protect_temporary_file: (Since version 5.0.12) Use this hook to remove protection for temporary files.
frm_entry_formatter_class: This filter allows changing the Entry formatter class to change how the entry is displayed.
frm_prepend_and_or_where: This filter allows changing the whole or a part of the where clause in the database query. Note: This filter has many database queries call. Do as many as checks possible before changing the where clause to prevent unexpected issues.
frm_entry_formatter_format: By default, FrmEntryFormatter class supports some format values (array, json, plain_text_block, and table). This filter helps you add a new format value based on the format passed to FrmEntryFormatter class.
frm_entries_show_args: This filter allows modifying the arguments when showing an entry on the Entries page.
frm_formatted_entry_values_content: This filter allows changing how the entry is displayed without creating a new class.
frm_after_destroy_entry: This hook allows you to do something after an entry is deleted.
frm_before_duplicate_entry_values: Use this filter to change the values in a duplicated entry before it gets saved. It is used in auto-increment [auto_id] values when an entry is duplicated.
frm_check_file_referer: Use this filter to control whether or not a protected file will check the referer on download.
frm_protected_file_readonly_permission: Use this filter to to work around a Snuffleupagus security module rule that prevents certain chmod values.
frm_saved_errors: This filters the error data before showing a form, and that form has already been submitted before.
frm_new_post: This hook is used to modify the post before it is created.
frm_add_entry_meta: This hook allows you to make changes to a value saved to a field right before it is sent to the database.
frm_upload_folder: The files uploaded through Formidable go to your wp-content/uploads/formidable folder. This may be different depending on where you have set your regular file uploads to be stored. You can view the uploaded files in your WordPress Media Library, on the Formidable entries pages, or on any View you create. Use the filter if you would like to further organize your file uploads inside the "formidable" folder.
frm_delete_message: Use this hook to change the message shown after an entry is deleted from the front-end.
frm_redirect_url: Dynamically change the URL users are redirected to if more control is needed than the basic conditional statements allow, i.e. http://[if 25 equals="Option 1"]site-a.com[/if 25][if 25 equals="Option 2"]site-b.com[/if 25][if 25 equals="Option 3"]site-c.com[/if 25].
frm_is_field_hidden: This hook prevents validation on a reCaptcha field by indicating that it is hidden on the page.
frm_user_can_edit: This hook allows you to customize which users can edit and delete entries from the front-end.
frm_allow_delete: This hook can customize when an entry can be deleted. This hook is triggered right after frm_user_can_edit.
frm_update_entry: This hook allows you to do something with the data entered in a form before it is updated.
frm_pre_update_entry: This hook allows you to do something with the data that was entered in the form before it is changed and the form is updated.
frm_pre_create_entry: This hook can be used to manipulate the values in an entry prior to it’s creation.
frm_before_destroy_entry: This hook allows you to do something before an entry is deleted.
frm_time_to_check_duplicates: This hook can be used to change the amount of time that is allowed between duplicate entries. By default, duplicate entries can't be submitted within 60 seconds of each other. We recommend using this hook to fix the error message, It looks like you've already submitted that.
frm_create_cookies: By default, a cookie is created when a form is submitted that is setup to be limited by the cookie time. This hook allows you to prevent the cookies from being created.
frm_after_duplicate_entry: This hook allows you to something after an entry has been duplicated.
Customize the Email Notification
frm_to_email: This hook gives you added control over emails. You may customize it to send emails to specific recipients based on certain field selections or to conditionally stop the email from sending.
frm_notification_attachment: Use this hook to attach files to email notifications. The file must be uploaded to your site before it can be included in the email. All variations of this hook require the absolute path to the file. In WordPress, ABSPATH will give you the path to the root of your site. In most cases, your path will look like this: ABSPATH . '/' . 'wp-content/uploads/2015/02/filename.pdf'
Use frm_display_[field type]_value_custom hook instead
Change or format a value in the email notification before sending. This allows you to modify specific values in the email notification without modifying the entire email message.frm_send_separate_emails: Use this hook to send a separate email for each recipient in your email action's To box.
frm_rich_text_emails: Use this hook for cases when a plain textarea may be preferred over rich text.
frm_email_subject: This hook allows you to customize your email subject.
frm_email_message: This hook can be used to edit or replace the message body of an email sent by a form.
frm_send_email: This hook is used to stop an email from sending based on the content on the email.
Form Actions
frm_email_header: Customize headers for your email notifications.
frm_use_embedded_form_actions: Use this hook to determine whether the actions are triggered for an embedded form. By default, actions are not triggered for embedded forms.
frm_email_control_settings: This hook can be used to manipulate the email settings of a form.
frm_action_logic_value: Make adjustments to the value used in form action logic. This filter is run each time a row of logic is evaluated at the time a form action is triggered.
frm_skip_form_action: Use this hook to conditionally skip Form Actions. Limitation: It is not intended to work with form actions triggered by the Form Action Automation add-on.
frm_api_post_response: This hook allows you to do something with the response returned after an API call.
frm_form_action_limit: This hook allows you to manipulate the limit of the form actions. There is a limit of 99 actions on a single form by default.
frm_api_request_args: This hook allows you to do something with the request returned after an API call.
frm_get_met_on_submit_actions: Use this hook to filter the On Submit actions that meet the conditional logic.
frm_get_run_success_action_args: Use this hook to filter the run success action args.
frm_redirect_delay_time: Use this hook to filter the delay time before redirecting when On Submit Redirect action is delayed.
frm_create_default_email_action: Use this filter to disable the email action added by default when a new form is created.
frm_create_default_on_submit_action: Use this filter to disable the confirmation action added by default when a new form is created.
frm_on_submit_action_options: Use this hook to surpass the default limit of 99 when adding On Submit actions
frm_pro_repeater_action_support: Use this filter hook to allow adding or removing repeater actions support for form action type.
frm_before_action_settings: This action hook fires before any form action settings.
frm_custom_trigger_action: This filter hook allows triggering custom actions, and skipping the default trigger.
Display Data
frm_where_filter: Use this hook to customize a filter for a View. This hook will only apply to filters you have added in the "Advanced Settings" section of your View. Please note that this hook is not designed to work with post fields or custom fields. A full database call is necessary to make this work with post or custom fields.
frmpro_fields_replace_shortcodes: This hook allows you to change the value displayed in emails, Views, or anywhere else that Formidable shortcodes are accepted.
frm_graph_value: This hook allows you to change the values used in the graphs.
frm_show_entry_dates: This hook allows you to customize the dates where an entry will be displayed when using a calendar View. Use this hook to add recurring events to a Calendar View.
frm_filter_where_val: Use this hook to customize a filter for a View. This hook will only apply to filters you have added in the "Advanced Settings" section of your View. This hook is similar to the frm_where_filter hook, but is fired earlier and changes only the value used before it is used in the full database query.
frm_search_for_dynamic_text: Use this hook to search numeric values in a Dynamic field. Normally, numeric values are treated as entry IDs in Dynamic fields so the database call will look for matching entry IDs rather than searching the linked field's values. This hook will will be used specifically for Dynamic field filters added in a View.
frm_filter_view: The frm_filter_view hook allows you to modify any part of the View object before the View is rendered.
frm_search_any_terms: Use this hook to search for an entire string with the Search bar. Normally, results will be returned that match any of the searched words.
frm_before_day_content: This hook can be used to add content at the beginning of a calendar day, before the entries are listed.
frm_display_[field type]_value_custom: Adjust the standard value shown in the email, entries page, or views. The name of the hook will changed based on the type of field being displayed.
frm_views_fields_in_create_view_popup: This hook can be used for customizing the checkbox options when creating a new Table View.
frm_graph_id: Use this filter to customize the ID used for graphs.
get_canonical_url: Use this filter to customize the canonical URL for a page/post.
frm_display_inner_content_before_add_wrapper: Use this hook to allow filtering the inner content of a grid view before the wrapper element is added.
frm_views_table_class: Use this hook to allow filtering the table view CSS class.
frm_filter_final_view: Use this filter to modify the final result of a View.
frm_display_value: This hook can be used to adjust the displayed values.
frm_no_data_graph: Use this hook to edit the "No Data" message that appears when there is nothing to display in a graph.
frm_filter_auto_content: Use this hook to prevent automatic filtering of a view used on a single post or page.
frm_google_chart: Use this hook to add or change the options in your Google chart.
frm_view_order: This hook allows you to manipulate how a view is ordered.
frm_graph_data: This hook will allow you to manipulate all the data in a graph, including the labels, rows, and columns.
frm_before_display_content: Use this hook to customize the Before Content of a View.
frm_after_display_content: Use this hook to customize the After Content section of a View.
frm_display_entry_content: Use this hook to customize content in your View, like adding a row counter.
frm_display_entries_content: Use this hook to customize content in your View.
frm_no_entries_message: Use this hook to customize the "No Entries" message for a View.
CSV and XML Management
frm_map_csv_field: This hook can be used to automatically match the headers in your .csv file with the fields in your form.
frm_after_import_form: This hook is triggered immediately after importing an XML form, allowing you to make any changes to the form that was just imported, if needed, or perform other actions.
frm_csv_sep: This hook can be used to manipulate the separator for checkboxes and other arrays in a .csv file.
frm_csv_line_break: This hook allows you to manipulate the line breaks in an .csv file.
frm_csv_date_format: This hook allows you to manipulate the format of date fields in an .csv file.
frm_default_templates_files: The default templates are generated and updated when the Formidable database number increases. So almost every time you update Formidable, the default templates are also updated.
frm_csv_format: By default, you can set the CSV export format when exporting on the Import/Export page. However, exporting from the entries page defaults to UTF-8. This hook can be used to change the format of the CSV exported from your entries page.
frm_csv_column_sep: This hook can be used to manipulate the column separator for a .csv file.
frm_editing_entry_by_csv: This hook can be used to filter the values of an entry in a csv import.
frm_xml_response: This hook can be used for altering the message/response when an XML file is imported.
frm_export_csv_headings: Use this filter to add, rename and remove a column from CSV export.
frm_fields_for_csv_export: This filter allows you to remove a specific field from the CSV export.
frm_xml_filename: Use this filter to customize the file name of a downloaded XML export from the Formidable → Import/Export page. The generated XML filename usually includes the site name on the front and the date at the end. e.g. example.formidable.2022-04-28.xml
frm_should_import_files: This filter expects a boolean true/false. If this filter is on, URLs in item meta for file fields will be uploaded to the site when importing files. When it's off, it won't. It is controlled by default with the "Import Files" checkbox on the import screen. However, in some cases, the import doesn't use that interface, but this filter still applies.
frm_csv_value: This hook can be used to manipulate or add a value to your .csv file.
frm_csv_columns: This hook can be used to manipulate the columns that appear in .csv files that are exported. Note: This filter does not work with the Export Views as CSV add-on, it only works for exporting the entries list CSV. If you are using the add-on, learn more about its list of available hooks.
frm_csv_filename: This hook can be used to customize your CSV filename when exporting entries from Formidable.
frm_csv_where: Use this hook to determine which entries will be exported when you export a CSV file.
frm_csv_headers: Use this hook to customize the header row(s) in your CSV export from the Formidable → Import/Export page.
Creating Add-ons
Add a new field: View examples for adding fields to the form builder.
frm_default_value_setting: Add extra settings in the 'Default Value' section in the field settings.
frm_after_field_options: Add settings at the bottom of the field options in the sidebar.
frm_field_options: Add settings in the field-specific section in field options.
frm_[type]_primary_field_options: Replace [type] in the hook with the name of the field type. This adds settings into the field-specific section in the field settings.
frm_[settings]_settings_form: Add settings or content on the Global settings page. The name of the tab will be the name of the hook. A few examples include:
- frm_general_settings_form
- frm_messages_settings_form
- frm_permissions_settings_form
- frm_misc_settings_form
frm_field_options_form: Use this hook to include extra options in the field options box. The additional options added using this hook will only appear in the admin settings and will not be saved in the database. Note: If you need to save the additional field options in the database, use the frm_field_options hook instead.